R Code Optimization
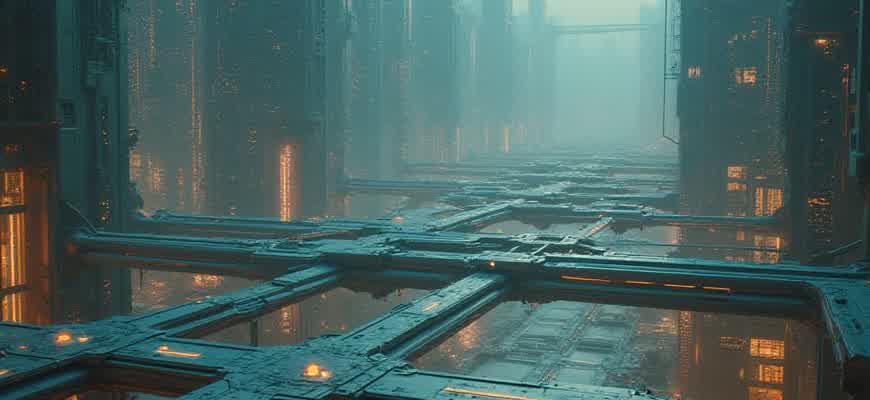
Improving the performance of R scripts is crucial for handling large datasets and complex computations. By refining the code, developers can achieve faster execution times and better resource management. Below are some common techniques to optimize R code:
- Vectorization - Replacing loops with vectorized operations that are native to R, significantly reducing computation time.
- Efficient Memory Usage - Managing memory allocation effectively to avoid unnecessary copies of data during computation.
- Profiling and Benchmarking - Identifying bottlenecks in the code using profiling tools such as Rprof and microbenchmark.
For more advanced optimizations, consider the following:
- Parallel Computing - Utilizing multicore processors or distributed systems to parallelize computations and enhance performance.
- Data Table Usage - Switching from data.frames to
data.table
for faster data manipulation and subsetting. - Using Efficient Packages - Leveraging libraries such as
Rcpp
anddata.table
that offer optimized operations.
Tip: Profiling is the first step in any optimization process. Always benchmark your code before and after making changes to ensure improvements.
Optimization Technique | Benefit |
---|---|
Vectorization | Faster computation, lower memory usage |
Parallel Computing | Improved speed for large-scale data processing |
Efficient Data Structures | Faster access and manipulation of data |
Identifying Performance Bottlenecks in R for Optimized Execution
Optimizing R code involves recognizing parts of your script that slow down the execution process. Performance bottlenecks can occur in various places such as inefficient loops, unoptimized data handling, or unnecessary computations. Identifying and addressing these issues is crucial for speeding up the analysis, especially when dealing with large datasets.
To effectively pinpoint the bottlenecks, it's important to use profiling tools and understand where the most time is being spent. By systematically narrowing down the problematic areas, you can apply specific techniques to enhance the performance of your code.
Techniques to Identify Code Bottlenecks
- Profiling with Rprof(): This tool helps to monitor the execution time of each function, allowing you to identify which sections of the code are taking the most time.
- Using system.time(): This simple function measures the time it takes to execute a particular expression, helping you compare different code approaches.
- Benchmarking with microbenchmark(): For more precise timing, microbenchmark allows comparison of multiple executions of an expression, showing which versions are faster.
- Visual Profiling Tools: Tools like profvis provide a graphical interface for viewing performance metrics and understanding where optimization is needed.
Common Bottlenecks in R Code
- Loops and Recursive Functions: Inefficient loops, especially in large datasets, can severely degrade performance. Vectorization is often a better approach.
- Large Data Structures: Storing and manipulating large datasets in memory can lead to excessive memory usage and slowdowns. Using memory-efficient packages like data.table or dplyr can help.
- Unnecessary Recalculation: Repeating calculations that don’t need to be recalculated wastes both time and resources. Use caching or store intermediate results when possible.
Example: Profiling a Slow Function
Here’s an example of using Rprof() to identify bottlenecks:
Rprof("profile.out") # Run the code you want to profile summaryRprof("profile.out")
The output will give you a detailed breakdown of where the time is spent, helping you target specific functions or processes that need optimization.
Optimizing Common Bottlenecks
Bottleneck | Optimization Tip |
---|---|
Loops | Use vectorized functions or apply family functions like lapply() to avoid the overhead of traditional for-loops. |
Large Data Manipulations | Leverage memory-efficient packages like data.table for faster data handling and manipulation. |
Repetitive Calculations | Cache results using memoise() or store intermediate results in variables to avoid redundant computations. |
Memory Management: Reducing Memory Usage in R Code
Efficient memory management is critical when working with large datasets in R, as it can significantly impact the performance and scalability of your analysis. Poor memory utilization leads to slower execution times, increased risk of crashes, and higher system resource consumption. By using memory-friendly coding practices, you can minimize these issues, ensuring smoother workflows and reduced overhead when processing large volumes of data.
To achieve optimal memory usage, it is important to consider how objects are stored in memory and how they interact with each other. Proper management of memory resources can be achieved through techniques such as removing unnecessary objects, using memory-efficient data structures, and making use of in-place operations when possible. Below are some strategies that can help optimize memory usage in R.
Key Strategies for Efficient Memory Usage
- Remove Unused Variables: After variables are no longer needed, use the rm() function to delete them from memory.
- Use Data Tables: Consider using data.table instead of data.frames for large datasets, as it is more memory-efficient and faster for manipulation tasks.
- Optimize Loops: Try to avoid growing objects inside loops, as this can cause R to repeatedly reallocate memory.
- Work with In-Place Modifications: Modify objects in-place rather than creating copies to avoid unnecessary memory consumption.
- Use Memory-Mapped Files: For extremely large datasets, memory-mapping techniques can be used to work with data that doesn’t fit into RAM.
Important Considerations for Memory Optimization
Memory usage can be dramatically reduced by avoiding creating multiple copies of large data objects. The copy() function in data.table, for example, should be used with caution as it generates a new object, consuming additional memory.
Recommended Memory-Efficient Data Structures
Data Structure | Memory Efficiency | Usage |
---|---|---|
data.table | Highly memory-efficient | Large datasets, fast manipulation |
matrix | Efficient for numerical data | Mathematical operations |
list | Good for storing heterogeneous data | Storing different types of objects |
In summary, reducing memory usage in R requires careful attention to the way data structures are managed and how objects interact with each other in memory. Implementing the above practices will help minimize unnecessary memory usage, improve performance, and prevent system overloads.
Utilizing Vectorized Operations for Enhanced Data Processing in R
In R, vectorization refers to the ability to perform operations on entire datasets at once, instead of using loops. This is crucial for improving performance when processing large datasets, as R’s internal vectorized functions are highly optimized for speed. Vectorized operations not only simplify the code, but they also significantly reduce execution time, especially when compared to iterative constructs like for-loops.
By leveraging vectorized functions, users can process and manipulate data in an efficient manner. R is designed to work with vectors, matrices, and arrays, allowing you to perform mathematical and logical operations directly on these data structures. This results in more concise and readable code while improving computational efficiency.
Key Advantages of Vectorization in R
- Speed: Vectorized operations can be hundreds or even thousands of times faster than traditional loops.
- Simplicity: The code becomes cleaner and easier to maintain.
- Memory Efficiency: Since R processes the entire vector at once, memory usage is optimized, especially with large datasets.
Examples of Vectorization in R
Below is a comparison between using loops and vectorized operations to calculate the square of each number in a vector:
# Using a loop
numbers <- 1:1000000
squares_loop <- numeric(length(numbers))
for(i in 1:length(numbers)) {
squares_loop[i] <- numbers[i]^2
}
# Vectorized approach
squares_vectorized <- numbers^2
While the loop performs the operation step-by-step, the vectorized approach directly computes the squares for all elements, resulting in faster execution time and cleaner code.
Tip: Use functions like apply(), sapply(), and lapply() for more complex tasks that require applying a function over vectors or lists.
Performance Comparison
Method | Execution Time |
---|---|
For Loop | ~10 seconds |
Vectorized Operation | ~0.01 seconds |
As demonstrated, vectorization leads to a remarkable reduction in processing time, making it a key optimization strategy for data-intensive tasks in R.
Improving Loop Performance in R: Alternatives to For Loops
When working with large datasets in R, using traditional for loops can become a bottleneck in performance. Although for loops are easy to implement and understand, they are often inefficient due to the overhead of repeatedly evaluating conditions and accessing data in each iteration. Several alternatives can be utilized to significantly improve the performance of your code.
One of the most effective methods for optimizing loops in R is replacing for loops with vectorized operations or using specialized functions from efficient packages. These alternatives leverage internal C code or optimized algorithms that reduce computational time significantly. Below are some common approaches to enhance loop efficiency.
Vectorization
Vectorization is one of the core principles for writing efficient R code. By applying operations directly to vectors or matrices, you eliminate the need for explicit loops. R performs these operations internally in a much faster and optimized manner.
Vectorized functions like sum(), mean(), and apply() operate over entire data structures, reducing the need for iteration and improving performance.
Using apply Family of Functions
Instead of using for loops, the apply family of functions such as lapply(), sapply(), and apply() can be used to apply a function to each element of a list or array.
- lapply(): Works on lists and returns a list.
- sapply(): Similar to lapply(), but attempts to simplify the result into an array or vector.
- apply(): Works on arrays or matrices, applying a function across rows or columns.
Table: Comparison of Looping Methods
Method | Typical Use Case | Performance |
---|---|---|
For loop | Iterating through elements one by one | Slow for large datasets |
Vectorization | Element-wise operations | Very fast |
Apply family | Matrix or list operations | Moderately fast, depending on data |
Utilizing R's Parallel Computing for Enhanced Scalability
Parallel computing in R provides significant improvements in processing large datasets and executing time-consuming tasks. By distributing work across multiple CPU cores or even different machines, R can handle more data and complex calculations efficiently. This capability becomes especially useful in computational tasks like simulations, statistical modeling, or machine learning, where tasks can be divided into smaller chunks for simultaneous execution.
Leveraging parallelism is essential for scaling applications in R, as it allows for better resource utilization and faster execution times. Various packages, such as `parallel`, `foreach`, and `future`, offer straightforward ways to implement parallel computation within R scripts. Understanding these tools and their appropriate usage is key to optimizing performance without overcomplicating the code.
Key Strategies for Parallelizing Code in R
- Use of `mclapply()` for Multicore Processing: This function from the `parallel` package is a simple way to execute functions in parallel using multiple cores on a single machine.
- Cluster-Based Parallelism with `makeCluster()`: This method allows users to create a cluster of worker nodes to handle different parts of a task, making it suitable for distributed computing across multiple machines.
- Task Parallelism with `foreach` and `doParallel`: This combination enables efficient handling of independent tasks in parallel, ideal for loops or large repetitive computations.
Best Practices for Scalability
- Minimize Data Transfer Between Nodes: To avoid bottlenecks, ensure that large datasets are distributed efficiently and avoid frequent communication between worker nodes.
- Use Efficient Data Structures: R's data structures such as data tables (e.g., `data.table`) or matrices are optimized for faster parallel processing compared to basic data frames.
- Monitor System Load: It's crucial to monitor CPU and memory usage to prevent overloading the system and ensure that the parallel tasks are well-distributed.
"By distributing tasks across multiple cores or machines, R’s parallel computing features allow for significant reductions in computation time, enabling the analysis of larger datasets and more complex models."
Example: Parallelizing a Computation Task
Code | Description |
---|---|
library(parallel) | Load the necessary library for parallel computing. |
cl <- makeCluster(detectCores() - 1) | Create a cluster using all available cores except one (for the main process). |
result <- parLapply(cl, 1:1000, function(x) x^2) | Distribute the task of squaring numbers from 1 to 1000 across the cluster. |
stopCluster(cl) | Stop the cluster after completing the computation. |
Optimizing Data Import and Export in R Projects
Efficient handling of data import and export is critical for improving the performance and scalability of R projects. Large datasets can lead to significant bottlenecks, especially when reading from or writing to files. Understanding the best methods and tools available for managing this process can drastically reduce runtime and memory usage. Additionally, the type of data and its format play a key role in optimization, influencing both the time and resources required for these tasks.
To ensure smooth data handling, various techniques can be employed. These methods involve using optimized functions and packages that minimize the overhead associated with I/O operations. Below are several practical approaches that can be applied when working with large or complex datasets in R.
Key Techniques for Optimizing Data Handling
- Use Efficient File Formats: Choose binary formats like RData or feather instead of CSV or Excel files to reduce read/write times.
- Leverage Data Table Packages: Use data.table or readr for faster and more memory-efficient data operations compared to base R functions.
- Optimize Data Types: Convert columns to the most efficient data types (e.g., factors instead of character strings) before exporting or processing large datasets.
Recommended Practices for Data Import
- Use fread() from data.table for fast CSV imports.
- For Excel files, consider using readxl or openxlsx as they are typically faster than the base read.csv() function.
- Minimize memory usage by selecting relevant columns during the import stage using options like colClasses or select.
Optimizing Data Export
Exporting data efficiently is just as important as importing it. The following approaches can help when saving data from R:
- Use write_fst() for fast binary storage in the fst format.
- Export to feather format for efficient transfer between R and Python.
- Minimize memory consumption: Write data in chunks if the dataset is extremely large, to avoid memory overload during the export process.
By using efficient file formats and specialized packages, you can significantly speed up both the import and export processes in your R projects, especially when dealing with large datasets.
Summary of Key Tools
Package/Method | Use Case | Speed |
---|---|---|
fread() | Fast import of CSV files | High |
write_fst() | Efficient export in binary format | High |
readxl | Read Excel files | Moderate |
Profiling R Code: How to Detect and Fix Slow Functions
When working with R, performance issues can arise in various forms, often slowing down the execution of functions and impacting the overall efficiency of data analysis. To optimize the performance, it's essential to identify the specific functions that consume the most time. Profiling is a crucial step in this process, allowing developers to pinpoint the bottlenecks in their code and address them accordingly.
By utilizing R's built-in profiling tools, you can examine how long each part of your code takes to execute. These insights are critical for deciding which functions need optimization. Profiling tools like `Rprof` and `system.time()` give a detailed breakdown of function execution times, which can highlight performance issues in large or complex datasets.
Profiling Techniques in R
To identify slow functions, you can use the following methods:
- Rprof() - This function provides detailed information about function calls, including time spent on each function.
- system.time() - This function measures the time it takes for a single expression or function to execute.
- microbenchmark - A package that benchmarks small sections of code to give you a precise measurement of performance.
Steps to Optimize Slow Functions
Once you've identified the slow functions, here are some strategies for improving their performance:
- Vectorization: Convert loops into vectorized operations, which are typically much faster in R.
- Efficient data structures: Use efficient data structures such as matrices or data tables instead of data frames for large datasets.
- Parallel processing: Leverage multiple cores of your processor to run code concurrently using the parallel or future packages.
- Memory management: Be mindful of memory usage. Avoid unnecessary copies of large datasets by using data.table or ff for large data.
Important Considerations
It’s crucial to regularly profile your code during development, especially when working with large datasets, as performance bottlenecks can be difficult to detect until they become problematic.
Example: Profiling and Optimizing a Slow Function
Function | Original Execution Time | Optimized Execution Time |
---|---|---|
Loop over data frame | 15 seconds | 3 seconds |
Vectorized operation | 12 seconds | 1 second |
By following these steps, you can dramatically improve the performance of your R code and handle large datasets more effectively.
Choosing the Right R Libraries for Optimal Performance
When working with large datasets or complex statistical models in R, selecting the appropriate libraries can significantly enhance the performance of your analysis. R provides a vast range of packages, each designed with specific use cases in mind, from data manipulation to machine learning. Choosing the right one depends on your task requirements, the size of your data, and the computational resources available.
Understanding the underlying performance characteristics of each library is key. For example, some libraries are optimized for speed and memory efficiency, while others provide ease of use and versatility. The balance between these factors will determine how effectively you can handle your data and perform computations.
Key Considerations When Selecting R Libraries
- Task Requirements: Understand the core functionality of each package to align it with your specific needs. For example, libraries like data.table and dplyr are excellent for data manipulation, but data.table tends to be faster with larger datasets.
- Memory Management: Some libraries, such as bigmemory, are optimized for handling large datasets by minimizing memory usage.
- Parallel Computing: If your analysis involves intensive computations, consider libraries like parallel or future for parallel processing to speed up computations.
Popular Libraries for Enhanced Performance
Library | Use Case | Performance Characteristics |
---|---|---|
data.table | Data manipulation and aggregation | Fast, memory efficient, optimized for large datasets |
dplyr | Data wrangling and transformation | Readable syntax, good performance on small to medium datasets |
bigmemory | Handling large matrices and datasets | Memory efficient, works with large datasets on disk |
parallel | Parallel computing | Improves performance on multi-core systems |
Tip: Always benchmark different libraries with your specific dataset to determine which one provides the best performance for your use case.