Highway Traffic Github
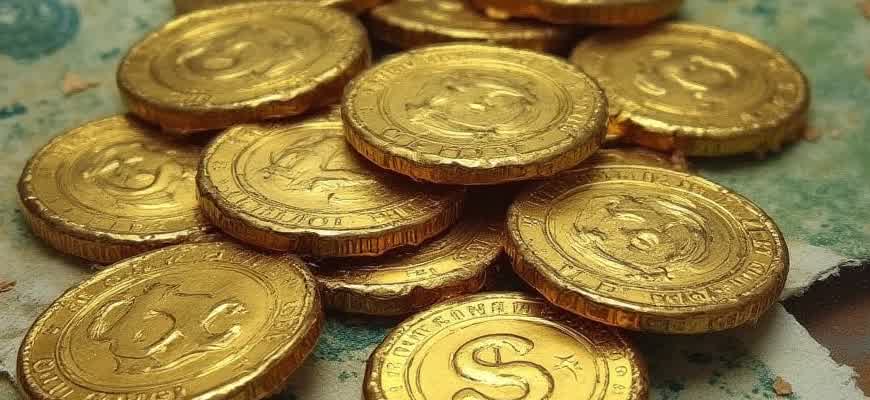
The management of highway traffic systems has become an essential aspect of urban planning, focusing on reducing congestion, improving safety, and optimizing traffic flow. Various tools and frameworks are available on platforms like GitHub to help developers and researchers in building intelligent systems for traffic monitoring and management.
Key Features of Traffic Management Projects on GitHub:
- Real-time traffic monitoring
- Incident detection and response systems
- Optimization algorithms for traffic light control
- Data visualization tools for traffic analysis
"GitHub hosts a variety of open-source projects that provide innovative solutions to modern traffic issues, contributing to smarter urban mobility."
These projects often utilize advanced technologies such as machine learning, sensor networks, and big data analytics to address key challenges in traffic management. The collaborative nature of GitHub allows for continuous development and improvement of these systems by global contributors.
Common Technologies in Traffic Management Repositories:
Technology | Purpose |
---|---|
IoT Sensors | Collect real-time traffic data |
Machine Learning | Predict traffic patterns and optimize control systems |
Big Data | Analyze and store vast amounts of traffic-related data |
Integrating Traffic Data into Your Github Repository
When managing highway traffic data on a Github repository, it’s essential to structure and handle the data efficiently. The integration process typically involves uploading datasets, establishing workflows, and enabling collaborative development. With the right approach, you can make the data accessible to developers and researchers working on related traffic or transportation projects.
This guide will walk you through key steps in the process, from preparing data files for upload to setting up automation tools. You’ll also learn about version control for large traffic datasets, ensuring data consistency, and enabling easy updates.
Steps to Upload Traffic Data to Your Repository
- Ensure your data is cleaned and formatted. Common formats include CSV, JSON, or XML.
- Use Git LFS (Large File Storage) for large traffic datasets to avoid repository size limits.
- Commit and push the data files to the appropriate repository folder.
Creating an Automated Data Pipeline
For dynamic data updates, you can set up an automated pipeline to pull real-time or periodic traffic data from external APIs. This will keep your repository up-to-date without manual intervention.
- Choose a traffic data API that fits your needs (e.g., Waze, Google Traffic).
- Create a script (e.g., Python, Node.js) that fetches data from the API.
- Set up a GitHub Action or cron job to run the script periodically.
Traffic Data Version Control
Handling large datasets requires careful attention to version control. Here are some tips:
Best Practice | Description |
---|---|
Data Snapshots | Store each dataset update as a separate version to track changes over time. |
Use Git LFS | For large datasets, Git LFS helps keep your repository size manageable by storing the actual data in a separate location. |
Document Changes | Use detailed commit messages to explain any major changes or updates to the data. |
Important: Always back up traffic data before performing any large updates or integrations to avoid losing valuable information.
Optimizing Your Codebase for Real-Time Traffic Data Updates
Efficient handling of real-time traffic data requires careful optimization of your codebase to ensure responsiveness, scalability, and minimal latency. In high-traffic scenarios, it’s essential to use performant algorithms and data structures that can handle frequent updates without degrading system performance. A well-structured codebase facilitates better maintenance and easier integration with external traffic data sources, which is critical for long-term sustainability.
To enhance the real-time performance of your system, consider using techniques that allow the code to scale seamlessly under varying loads. This involves optimizing both the server-side processing and the communication layer to quickly update traffic data while minimizing unnecessary resource consumption.
Key Considerations for Optimization
- Data Caching: Reduce the frequency of external data calls by implementing a caching mechanism for traffic data that updates at a reasonable interval.
- Event-Driven Architecture: Use event-driven models to process updates only when new data is available, minimizing system overhead.
- Concurrency Management: Leverage multi-threading or asynchronous processing to handle concurrent data updates efficiently.
Best Practices for Code Optimization
- Minimize API Calls - Use batch requests or aggregate data to reduce the number of interactions with traffic data providers.
- Optimize Data Structures - Use efficient data structures (like hash maps) to quickly store and retrieve traffic data based on key attributes (e.g., location, timestamp).
- Leverage Message Queues - Use message queues (like Kafka or RabbitMQ) to decouple data ingestion and processing, ensuring better load distribution and failure recovery.
By following these guidelines, your system will be better equipped to handle large-scale real-time traffic data, providing accurate and timely updates to users with minimal delay.
Performance Metrics
Metric | Ideal Value | Performance Impact |
---|---|---|
Latency | Under 100ms | Reduced delay in data updates |
Throughput | 1000 updates/second | Ensures system can handle large traffic loads |
CPU Usage | Under 80% | Maintains system responsiveness |
Leveraging Highway Traffic APIs for Effective Data-Driven Development
Highway traffic data plays a crucial role in various applications ranging from traffic management to autonomous vehicle systems. By utilizing specialized traffic APIs, developers can access real-time and historical data that enables the creation of smarter, data-driven solutions. These APIs provide valuable insights into traffic flow, congestion patterns, accident reports, and road conditions, all of which are essential for optimizing transportation systems.
Incorporating traffic-related data into development processes enhances the ability to predict traffic congestion, optimize routing algorithms, and improve urban planning initiatives. By utilizing this information, developers can create applications that not only enhance the user experience but also contribute to more sustainable and efficient transportation systems.
Benefits of Using Traffic APIs in Development
- Real-Time Traffic Updates: Provides instant access to current road conditions, helping users avoid traffic jams and optimize routes.
- Accurate Predictions: Historical data helps predict future traffic patterns and accidents, enhancing route planning algorithms.
- Incident Alerts: Developers can integrate real-time accident data to alert users about potential hazards on their routes.
Applications of Traffic Data
- Smart City Planning: Data can be used for designing better urban road systems that reduce congestion.
- Autonomous Vehicles: Traffic data is vital for the development of self-driving cars, allowing them to navigate complex environments safely.
- Navigation Systems: Traffic APIs enhance the efficiency of GPS apps by providing up-to-date road conditions.
Key Considerations When Integrating Traffic Data
When integrating highway traffic data into an application, it is essential to ensure data accuracy and timeliness. Inaccurate data could lead to incorrect predictions and route suggestions, potentially causing delays or dangerous situations.
API Feature | Benefits |
---|---|
Traffic Flow Data | Helps predict congestion and optimize routing |
Incident Reports | Alerts users to accidents and hazardous conditions |
Historical Data | Used for traffic trend analysis and future predictions |
Setting Up Automated Traffic Monitoring with Github Actions
In modern software development, automating the monitoring of traffic on highway systems has become an essential task for many teams. With the help of tools like Github Actions, developers can streamline the process of monitoring traffic flow, detecting anomalies, and responding in real-time. By integrating automated traffic analysis into the CI/CD pipeline, teams can ensure quicker reaction times and improve overall system efficiency.
Github Actions provides a powerful platform for automating various tasks, including traffic monitoring. Through the creation of custom workflows, developers can automate traffic data collection, process analytics, and trigger alerts based on specific conditions. This guide will explore the steps involved in setting up automated traffic monitoring using Github Actions, focusing on the essentials of workflow creation, configuration, and integration with traffic APIs.
Steps to Set Up Automated Traffic Monitoring
- Create a Github Repository: Start by setting up a repository on Github to store your traffic monitoring scripts and configuration files.
- Define Workflow in Github Actions: In the repository, create a `.github/workflows` folder and define a YAML file that specifies your automated traffic tasks.
- Install Necessary Dependencies: Use actions like `actions/setup-python` to set up the required environment for your traffic monitoring script.
- Connect to Traffic Data Sources: Utilize APIs from traffic monitoring services or set up your custom data collection system.
- Set Monitoring Rules: Define specific conditions (e.g., high traffic volume, sudden traffic spikes) and create appropriate triggers or alerts for them.
- Monitor and Respond: After configuring the workflow, let the automation run at scheduled intervals and monitor results for anomalies.
Workflow Example
Below is a simplified example of a Github Actions workflow configuration to automate traffic data monitoring:
name: Traffic Monitoring Workflow on: schedule: - cron: '0 * * * *' # Runs every hour jobs: traffic-monitoring: runs-on: ubuntu-latest steps: - name: Checkout repository uses: actions/checkout@v2 - name: Set up Python uses: actions/setup-python@v2 with: python-version: '3.x' - name: Install dependencies run: pip install -r requirements.txt - name: Run traffic monitoring script run: python traffic_monitor.py
Monitoring Results and Alerts
Once the workflow is set up and running, it’s essential to monitor the results and set up appropriate alerts:
- Email Notifications: Send email alerts when traffic spikes or abnormal patterns are detected.
- Slack Integration: Push real-time alerts to Slack channels for immediate action.
- Data Logging: Record traffic data to a database for historical analysis.
"Automated monitoring ensures that no critical traffic issues go unnoticed, and response times are significantly reduced."
Table of Common Github Actions for Traffic Monitoring
Action | Description |
---|---|
actions/checkout | Checks out your repository so that the workflow can access its files. |
actions/setup-python | Sets up the Python environment needed to run traffic scripts. |
actions/upload-artifact | Uploads logs or reports generated by your traffic monitoring script. |
Handling Large-Scale Traffic Datasets in Your Github Project
When managing traffic data at scale, GitHub repositories can quickly become inefficient if proper strategies aren’t implemented. Datasets that exceed the standard file size limit (100 MB per file) need careful handling to prevent disruptions to the workflow. Moreover, working with large datasets often involves multiple users, which increases the complexity of maintaining version control and collaboration.
To avoid issues, consider breaking down the data into smaller chunks or using external storage solutions. Leveraging Git Large File Storage (LFS) is one common method to store and manage large binary files efficiently while keeping the repository itself light and fast to navigate.
Best Practices for Storing and Handling Traffic Data
- Use Git Large File Storage (LFS): Store large files like traffic data in Git LFS to manage versions of large files while keeping your main repository fast.
- Split the Datasets: Break down the dataset into smaller, more manageable files, ideally by time periods, geographical regions, or traffic types.
- External Data Hosting: For extremely large datasets, consider using platforms like AWS S3, Google Cloud Storage, or even specialized databases for traffic data.
- Data Compression: Compress data files in formats like CSV.gz or Parquet to reduce the overall file size before uploading to GitHub.
Version Control Considerations
In large-scale traffic data projects, version control becomes more complex due to the size and frequency of changes to the datasets. To minimize performance bottlenecks, it’s critical to carefully structure the workflow:
- Use Separate Branches for Data Updates: Isolate major updates or modifications to the data in separate branches, reducing the burden on the main repository.
- Tagging and Releases: Use tags and release notes for datasets to ensure that different versions of the data can be easily tracked and retrieved.
- Incremental Updates: Rather than pushing full datasets, consider pushing only the incremental updates to minimize repository size and improve performance.
Important: For datasets that are frequently updated, always make sure to have a backup strategy in place. Using a cloud service for the storage and versioning of large files can ensure that you don't lose critical data during the development process.
Example Table for Traffic Dataset Breakdown
Region | Dataset Size | File Format | Compression |
---|---|---|---|
Urban Area | 2 GB | CSV | CSV.gz |
Highway | 3 GB | Parquet | Parquet (compressed) |
Rural Area | 1.5 GB | JSON | None |
Collaborating on Traffic Data Projects: Best Practices for Teamwork
When working on traffic data projects, collaboration is key to success. Whether it’s sharing datasets, developing algorithms, or analyzing traffic patterns, teamwork is essential. A well-coordinated team can help manage large datasets, identify important traffic trends, and create solutions that improve road safety and efficiency. The ability to effectively communicate and collaborate ensures that the project progresses smoothly and produces high-quality results.
To ensure the success of a traffic data project, it’s important to follow best practices for teamwork. This includes defining roles clearly, ensuring transparency in data handling, and using the right tools for collaboration. Proper documentation and version control are also critical to prevent confusion and ensure that all team members are on the same page.
Key Best Practices for Effective Collaboration
- Clear Role Assignment: Assign specific roles to each team member based on expertise. For example, one person may be responsible for data collection, another for analysis, and another for visualization or reporting.
- Frequent Communication: Regular meetings and check-ins are essential for aligning progress and discussing challenges.
- Version Control: Use GitHub or similar tools to manage changes to the codebase and track progress. This reduces the risk of conflicts and confusion when working on shared resources.
Effective Tools for Traffic Data Collaboration
- GitHub: Essential for version control and collaboration, allowing multiple contributors to work on the same codebase without issues.
- Jupyter Notebooks: Ideal for sharing code along with real-time analysis and visualizations, which is especially useful for traffic data projects.
- Slack/Teams: These tools help in maintaining communication, sharing updates, and quickly resolving issues within the team.
Best Practices for Data Management
Ensure that all data is clean, well-documented, and easily accessible for all team members. Having a clear naming convention and structured file system can save time and prevent errors.
Practice | Description |
---|---|
Data Versioning | Track changes to the dataset, ensuring every modification is recorded and traceable. |
Documentation | Provide clear instructions on how to interpret and use the data, as well as any assumptions or transformations made. |
Regular Backups | Ensure that data is regularly backed up to avoid potential data loss during the project lifecycle. |
Securing Your Highway Traffic Data with Github Security Features
When managing highway traffic data through a version control system, it's critical to ensure the data's confidentiality, integrity, and accessibility. GitHub, a popular platform for version control, offers several security features designed to protect your projects. By leveraging these tools, you can prevent unauthorized access, ensure proper version tracking, and safeguard sensitive data related to highway traffic systems.
In this context, GitHub provides various layers of security that enhance project management and protect data. These features allow you to set permissions, monitor activity, and secure sensitive information in your repositories, ensuring that only authorized personnel can make changes or access critical traffic data.
Key Security Features for Highway Traffic Data
- Branch Protection Rules: Set rules to enforce specific checks on code before merging, reducing the risk of introducing errors into the traffic data.
- Two-Factor Authentication (2FA): Add an extra layer of security by requiring users to verify their identity via a second authentication method when accessing sensitive data.
- Secret Scanning: Prevent accidental exposure of sensitive information, such as API keys or traffic system credentials, by scanning commits for secrets.
- Access Control: Define specific permissions for different collaborators, ensuring that only trusted individuals can make changes to the data.
Steps to Protect Your Highway Traffic Data
- Enable two-factor authentication for all users with access to the repository.
- Configure branch protection rules to require code review and successful checks before merging changes.
- Set up secret scanning to identify sensitive data in commits.
- Review and update access control settings to restrict permissions based on roles.
Note: Always be proactive in reviewing and updating your security settings to address new threats or vulnerabilities in the system.
Useful GitHub Security Tools
Feature | Description |
---|---|
Dependabot Alerts | Automatically detects vulnerabilities in your dependencies and provides recommendations for updates. |
Security Advisories | Create and share advisories regarding vulnerabilities in your projects to notify others of potential risks. |
GitHub Audit Logs | Track actions taken on repositories, providing a clear record for security monitoring. |
Showcasing Traffic Analytics Dashboards Using GitHub Pages
GitHub Pages provides an efficient and cost-effective platform for deploying web-based projects, making it ideal for hosting real-time traffic data visualizations. Developers can leverage this feature to present detailed dashboards, offering a comprehensive look into highway traffic patterns, trends, and insights. These dashboards can be dynamically integrated with traffic analytics tools and datasets to provide meaningful information for users.
By utilizing GitHub Pages, developers can create static websites that display interactive charts, graphs, and data tables. These visualizations can be enhanced with JavaScript libraries like D3.js or Chart.js to ensure a seamless user experience. Moreover, GitHub's version control capabilities allow for easy tracking of updates to traffic data and dashboards.
Key Benefits of Using GitHub Pages for Traffic Dashboards
- Cost-effective: GitHub Pages offers free hosting, making it an affordable option for small and large-scale traffic monitoring projects.
- Version control: Seamless integration with GitHub repositories allows for easy versioning of the traffic data and dashboards.
- Customizable: Using various front-end technologies, developers can tailor the dashboards to meet specific project requirements and provide a more engaging user interface.
Steps for Deploying a Traffic Analytics Dashboard on GitHub Pages
- Prepare the traffic data and analytics tools.
- Develop the dashboard using HTML, CSS, and JavaScript libraries.
- Push the files to a GitHub repository.
- Enable GitHub Pages in the repository settings and link to the main branch or a specific folder containing the dashboard.
- Publish and share the dashboard URL for public access.
GitHub Pages is an excellent solution for developers seeking a simple way to share dynamic traffic analytics dashboards without the complexity of traditional hosting platforms.
Example of Traffic Analytics Table
Location | Traffic Volume | Average Speed | Peak Time |
---|---|---|---|
Highway 1 | 12,500 vehicles/hour | 65 mph | 5:30 PM |
Highway 2 | 8,000 vehicles/hour | 55 mph | 7:00 AM |